Introduction to Python HTML Parsing
What is HTML Parsing?
HTML parsing is the process of analyzing a string of HTML code to identify its structure and extract relevant information. This involves breaking down the HTML into its constituent elements such as tags, attributes, and text content. HTML parsing is fundamental for web scraping, where the goal is to extract data from web pages, as well as for web automation and data analysis tasks.
Why Use Python for HTML Parsing?
Python is a popular choice for HTML parsing due to its simplicity, readability, and the rich ecosystem of libraries available for handling HTML. Here are a few reasons why Python stands out:
- Ease of Use: Python's syntax is clear and straightforward, making it accessible for beginners.
- Powerful Libraries: Libraries such as BeautifulSoup, lxml, and PyQuery provide robust tools for parsing and manipulating HTML.
- Community Support: Python has a large, active community, offering extensive documentation, tutorials, and forums for support.
- Integration with Other Tools: Python can easily integrate with other data processing and web scraping tools like Scrapy and Selenium, enhancing its capabilities.
If you are looking for expert Data Scraping Services, check below 👇
How to Parse HTML Using Python?
Parsing HTML in Python typically involves using one of the popular libraries: BeautifulSoup or lxml. Here’s a quick guide on how to use each:
Using BeautifulSoup
BeautifulSoup is a Python library for parsing HTML and XML documents. It creates parse trees from page source code that can be used to extract data easily.
Installation:
pip install beautifulsoup4
Basic Usage:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head><title>The Title</title></head>
<body>
<p class="title"><b>The Bold Title</b></p>
<p class="story">Once upon a time...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.title.string) # Output: The Title
Using lxml
lxml is another powerful library known for its speed and efficiency in parsing HTML and XML.
Installation:
pip install lxml
Basic Usage:
from lxml import html
html_doc = """
<html>
<head><title>The Title</title></head>
<body>
<p class="title"><b>The Bold Title</b></p>
<p class="story">Once upon a time...</p>
</body>
</html>
"""
tree = html.fromstring(html_doc)
title = tree.xpath('//title/text()')
print(title[0]) # Output: The Title
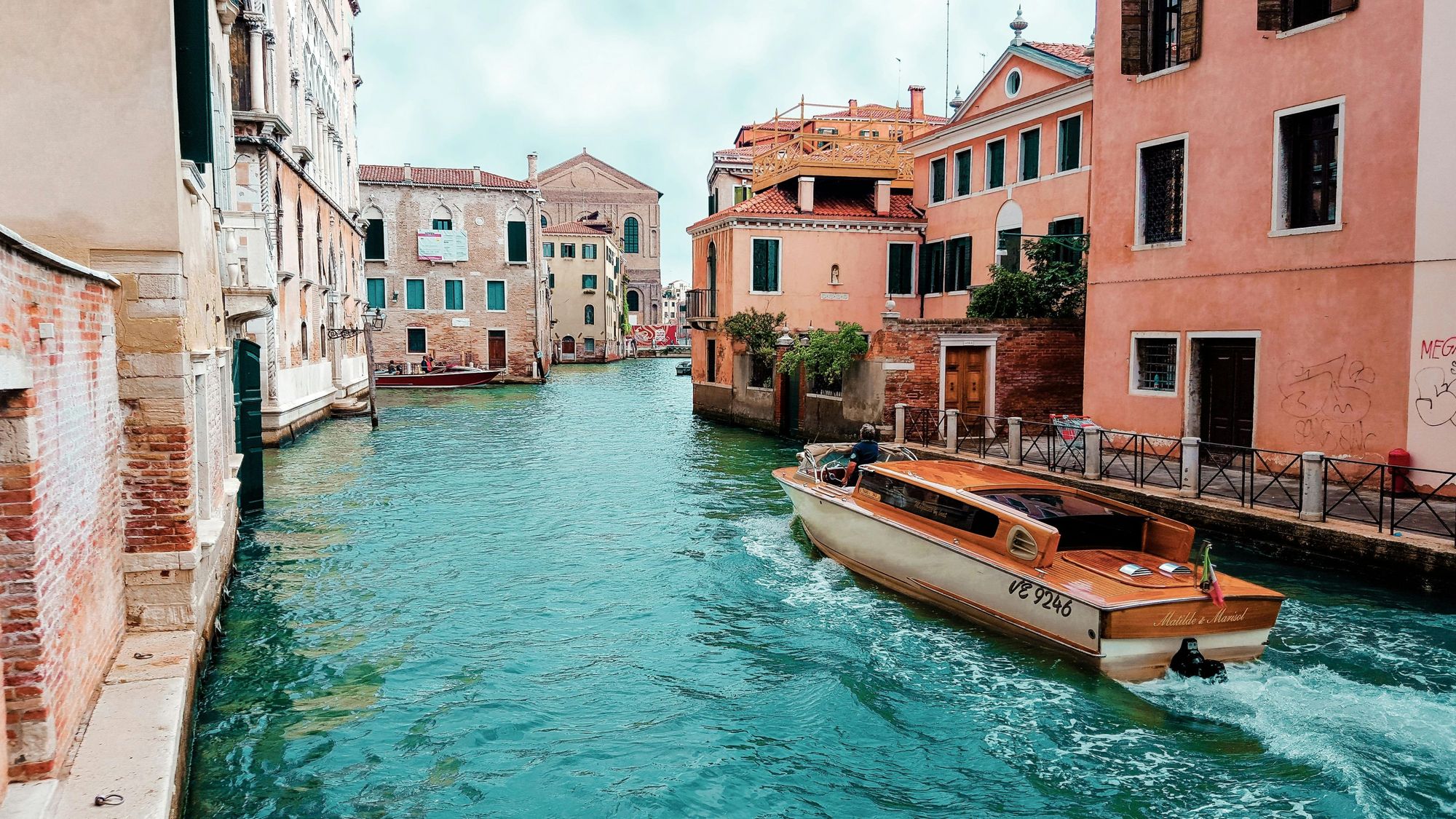
How to Read an HTML File Using Python?
Reading an HTML file in Python involves opening the file and parsing its content using a library like BeautifulSoup or lxml.
Example with BeautifulSoup:
from bs4 import BeautifulSoup
# Open and read the HTML file
with open('example.html', 'r', encoding='utf-8') as file:
html_content = file.read()
# Parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.title.string)
Example with lxml:
from lxml import html
# Open and read the HTML file
with open('example.html', 'r', encoding='utf-8') as file:
html_content = file.read()
# Parse the HTML content
tree = html.fromstring(html_content)
title = tree.xpath('//title/text()')
print(title[0])
How to Extract HTML Tags in Python?
Extracting HTML tags involves identifying specific elements within the HTML and retrieving their content or attributes.
Using BeautifulSoup:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head><title>The Title</title></head>
<body>
<p class="title"><b>The Bold Title</b></p>
<p class="story">Once upon a time...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
# Extract all paragraph tags
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.get_text())
# Extract all anchor tags and their href attributes
links = soup.find_all('a')
for link in links:
print(link['href'])
Using lxml:
from lxml import html
html_doc = """
<html>
<head><title>The Title</title></head>
<body>
<p class="title"><b>The Bold Title</b></p>
<p class="story">Once upon a time...</p>
</body>
</html>
"""
tree = html.fromstring(html_doc)
# Extract all paragraph texts
paragraphs = tree.xpath('//p/text()')
for p in paragraphs:
print(p)
# Extract all links and their href attributes
links = tree.xpath('//a/@href')
for link in links:
print(link)
What is the Best Python Library to Parse HTML?
Choosing the best Python library for HTML parsing depends on your specific needs:
- BeautifulSoup: Great for beginners due to its ease of use. It is flexible and easy to learn, making it ideal for quick scraping tasks.
- lxml: Offers faster performance and more powerful features compared to BeautifulSoup. It is suitable for handling large documents and complex parsing tasks.
- PyQuery: Provides a jQuery-like API, which can be advantageous for those familiar with jQuery. It is less commonly used than BeautifulSoup and lxml but still powerful.
For most use cases, BeautifulSoup and lxml are the go-to choices due to their robustness and extensive documentation.
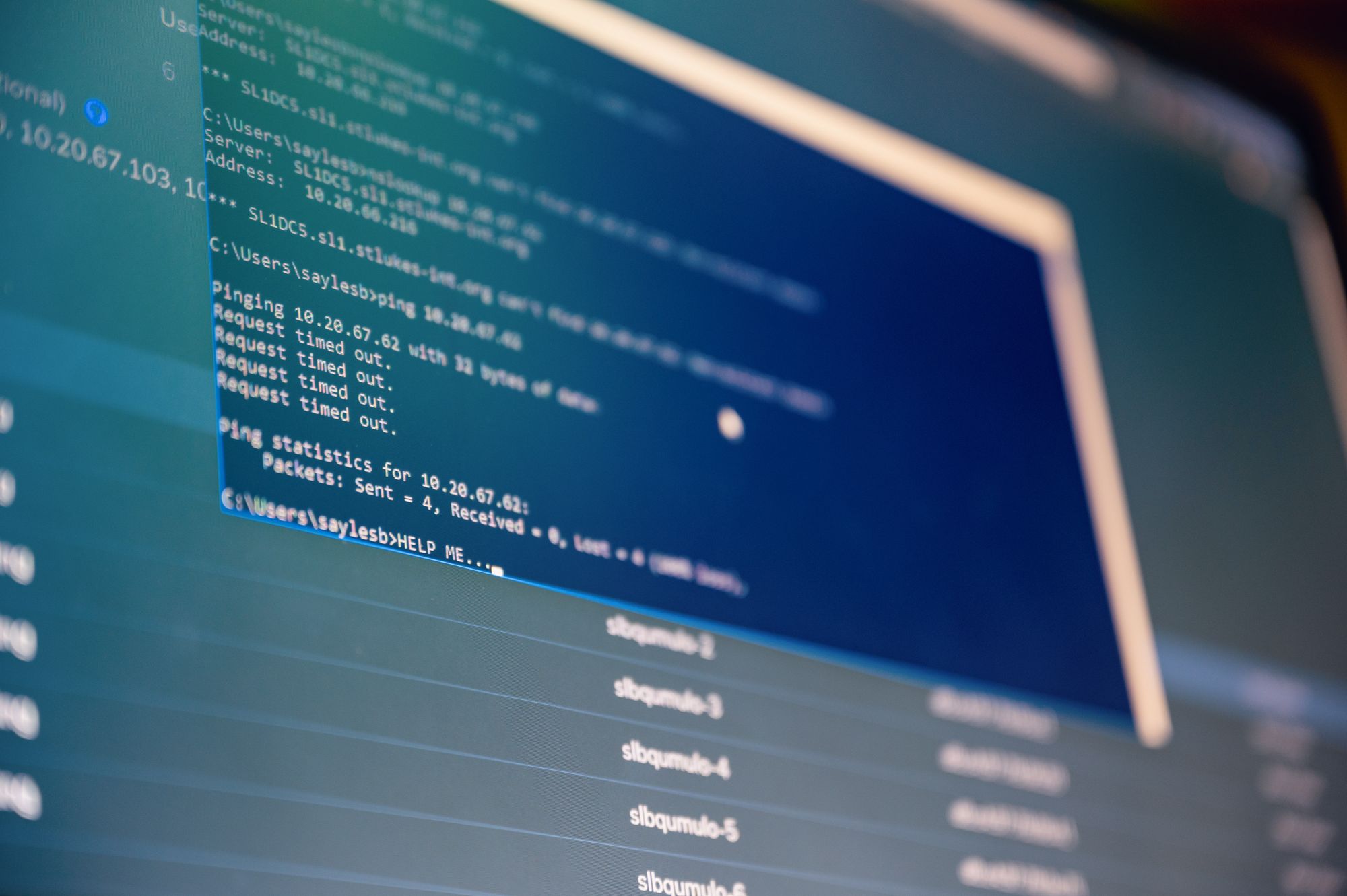
Getting Started with BeautifulSoup
Installing BeautifulSoup
Installing BeautifulSoup is straightforward using pip, the Python package installer.
pip install beautifulsoup4
pip install lxml # Optional, for faster parsing
Basic Usage Examples
Here are some basic examples to get you started with BeautifulSoup:
Example 1: Parse and print the title of a webpage
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.title.string)
Example 2: Extract all hyperlinks
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
Simple HTML Parsing Tasks
BeautifulSoup can handle a variety of simple parsing tasks such as extracting text, attributes, and navigating the parse tree.
Extracting all paragraph texts:
html_doc = """
<html>
<body>
<p class="title"><b>The Bold Title</b></p>
<p class="story">Once upon a time...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
paragraphs = soup.find_all('p')
for paragraph in paragraphs:
print(paragraph.get_text())
Navigating the parse tree:
html_doc = """
<html>
<body>
<p class="title"><b>The Bold Title</b></p>
<p class="story">Once upon a time...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
div = soup.div
print(div.p.get_text()) # Output: Story 1
Parsing HTML with lxml
Overview of the lxml Library
lxml is a powerful and efficient library for parsing HTML and XML in Python. It leverages the speed of the underlying C libraries, making it significantly faster than other parsing libraries like BeautifulSoup.
Differences Between BeautifulSoup and lxml
- Performance: lxml is faster due to its C implementation.
- Error Handling: BeautifulSoup is more forgiving with poorly formed HTML.
- Syntax: lxml uses XPath for querying, while BeautifulSoup uses a Pythonic API.
- Dependencies: lxml requires libxml2 and libxslt, which might need to be installed separately on some systems.
Examples of Using lxml for HTML Parsing
Example 1: Parse and print the title of a webpage
from lxml import html
import requests
url = 'http://example.com'
response = requests.get(url)
tree = html.fromstring(response.content)
title = tree.xpath('//title/text()')
print(title[0])
Example 2: Extract all hyperlinks
from lxml import html
import requests
url = 'http://example.com'
response = requests.get(url)
tree = html.fromstring(response.content)
links = tree.xpath('//a/@href')
for link in links:
print(link)
By following these guides and examples, you can leverage Python's powerful libraries to parse and manipulate HTML efficiently, whether you choose BeautifulSoup for its simplicity or lxml for its performance.
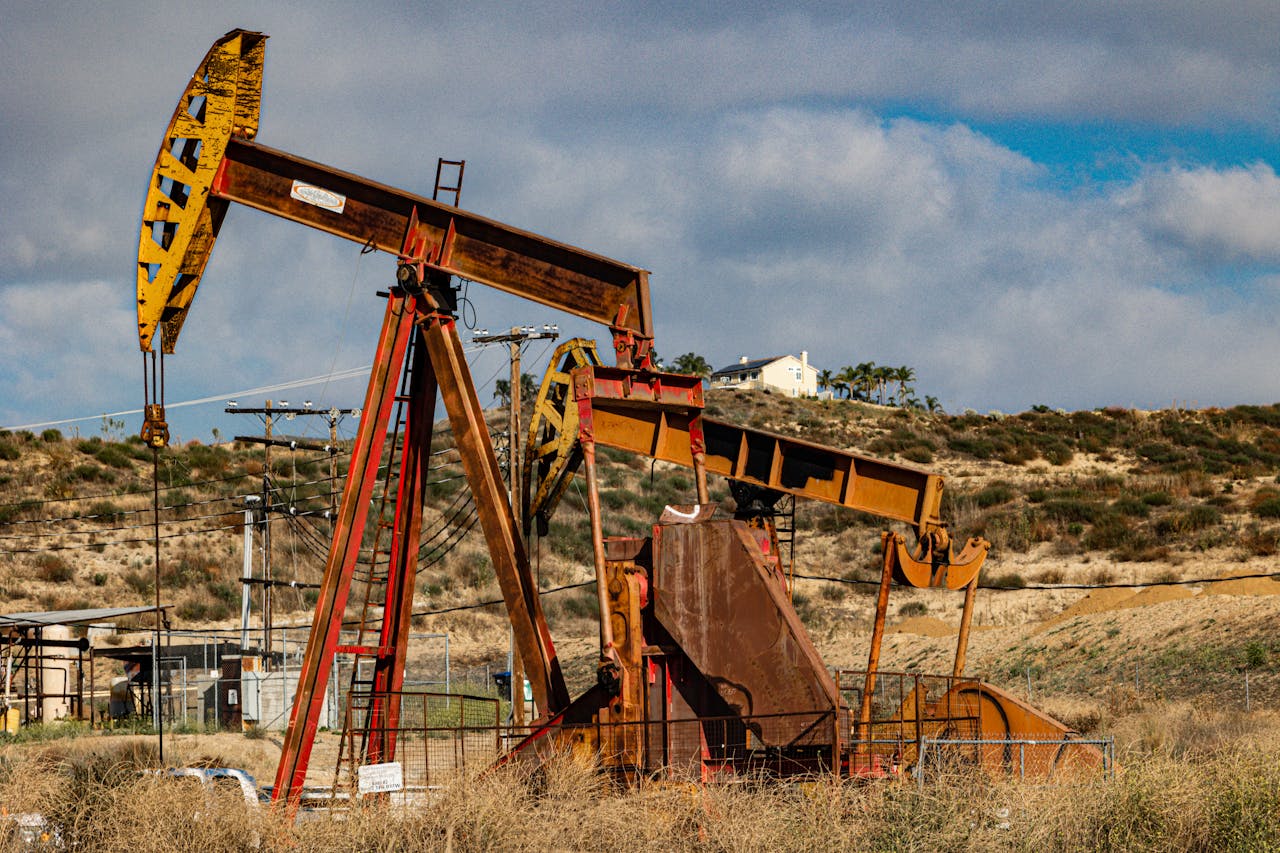
Elevate Your Data with DataHen! 🚀
Struggling with web scraping challenges? Let DataHen's expert  solutions streamline your data extraction process. Tailored for small to  medium businesses, our services empower data science teams to focus on  insights, not data collection hurdles.
👉 Discover How DataHen Can Transform Your Data Journey!